5. Commonsdialog Module¶
5.1. Main functions¶
- msgbox(message[, title="", meesageType=IDEA, root=None])¶
Shows a message dialog with ok button only.
- Parameters:
message (str) – text to present in the dialog
title (str) – title of the dialog
messageType (int) – type of icon to use.
root (DefaultFrame or None) – Frame reference
- inputbox(message[, title="", messageType=IDEA, initialValue="", root=None])¶
Shows a input dialog.
- Parameters:
message (str) – text to present in the dialog
title (str) – title of the dialog
messageType (int) – type of icon to use.
initialValue (str) – Initial value of the inputbox
root (DefaultFrame or None) – Frame reference
- Returns:
Return text in the input box
- Return type:
str
- confirmDialog(message[, title="", optionType=YES_NO, messageType=IDEA, root=None])¶
Create a message dialog with options button
- Parameters:
message (str) – text to present in the dialog
title (str) – title of the dialog
optionType (int) – bottons to show
messageType (int) – type of icon to use.
- filechooser(option[, title="", initialPath=None, multiselection=False, filter = None, fileHidingEnabled=True, root=None])¶
Allows configuration parameters to filechooser dialogs
- Parameters:
option (int) – file chooser selection mode. Allowed values: OPEN_FILE, OPEN_DIRECTORY, SAVE_FILE
title (str) – Window title
initialPath (str) – Initial path to the directory to open in the dialog
multiselection (boolean) – Allow select more than one object.
filter (List of Strings) – list of acepted extension files (“jpg”, “png”, “gif”)
fileHidingEnabled (boolean) – True if hidden files are not displayed
- Returns:
Selected path or list of paths
- openFileDialog([title='', initialPath=None, root=None])¶
Shows a window dialog to choose one file.
- Parameters:
title (str) – Window title. Default ‘’
initialPath (str) – Initial path to open in window dialog
- openFolderDialog([title='', initialPath=None, root=None])¶
Shows a window dialog to choose one folder.
- Parameters:
title (str) – Window title. Default ‘’
initialPath (str) – Initial path to open in window dialog
- saveFileDialog([title='', initialPath=None, root=None])¶
Shows a window dialog to choose one file.
- Parameters:
title (str) – Window title. Default ‘’
initialPath (str) – Initial path to open in window dialog
- getJavaFile(path)¶
Returns a java File using parameter path. If path doesn’t exists looks for user home folder and if can not find it, returns path will be gvSIG instance directory.
- Parameters:
path (str) – String-path.
- Returns:
Return java.io.File
5.2. Library constants¶
Constants appearing inside the commonsdialog module that we will use in different functions:
*messageType options*
FORBIDEN = 0
IDEA= 1
WARNING= 2
QUESTION= 3
*Confirmdialog optionType Options*
YES_NO = 0
YES_NO_CANCEL = 1
ACEPT_CANCEL = 2
YES = 0
NO = 1
CANCEL = 2
*filechooser options*
OPEN_FILE = 0
OPEN_DIRECTORY = 1
SAVE_FILE = 2
*filechooser selectionMode*
FILES_ONLY = JFileChooser.FILES_ONLY
DIRECTORIES_ONLY = JFileChooser.DIRECTORIES_ONLY
5.3. Use case¶
The commonsdialog module managers the popup windows inside gvSIG. For example, if we want to show a warning to the user, we will use a msgbox()
: function. If we want to ask to the user for a value, we could use the inputbox()
function with will return a to the script the value ready to be used as a parameter in the code.
To import commonsdialog:
import gvsig.commonsdialog
or:
from gvsig import commonsdialog
or:
from gvsig.commonsdialog import *
For example:
1from gvsig import commonsdialog
2
3def main(*args):
4
5 commonsdialog.msgbox("Welcome to gvSig","Welcome", commonsdialog.IDEA)
We establish the type of the message in the messageType
parameter as we can see in msgbox()
, all the type are stored as constants in the commonsdialog
module.
Also, it depends of how we have imported them.
1from gvsig.commonsdialog import *
2
3def main(*args):
4
5 msgbox("Bienvenido a gvSIG", "Welcome", IDEA)
The execution give us as a result this window:
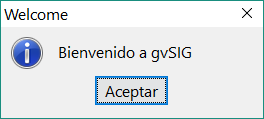
It depends of the message type how the icon of the window will be:
WARNING:
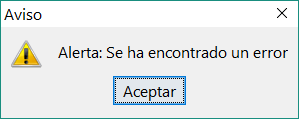
FORBIDEN:
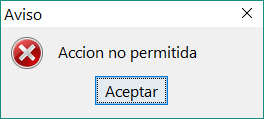
QUESTION:
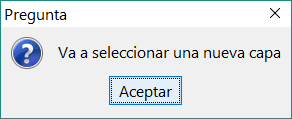
5.4. Dialog types¶
Differnt dialog types:
from gvsig import *
from gvsig import commonsdialog
from gvsig.commonsdialog import *
def main(*args):
message = "Test"
mb = commonsdialog.msgbox(message, title="", messageType=IDEA, root=None)
print "msgbox:", mb
ib = commonsdialog.inputbox(message, title="", messageType=IDEA, initialValue="", root=None)
print "inputbox:", ib
cd = commonsdialog.confirmDialog(message, title="", optionType=YES_NO, messageType=IDEA, root=None)
print "confirmDialog:", cd
option = "OPEN_FILE"
fc = commonsdialog.filechooser(option, title="", initialPath=None, multiselection=False, filter = None, fileHidingEnabled=True, root=None)
print "filechooser:", fc
fc = commonsdialog.filechooser(option, title="", initialPath=None, multiselection=True, filter = None, fileHidingEnabled=True, root=None)
print "filechooser:", fc
ofiled = commonsdialog.openFileDialog(title='', initialPath=None, root=None)
print "openFileDialog:", ofiled
ofolderd = commonsdialog.openFolderDialog(title='', initialPath=None, root=None)
print "openFolderDialog:", ofolderd
sfd = commonsdialog.saveFileDialog(title='', initialPath=None, root=None)
print "saveFileDialog:",sfd
Msgbox:
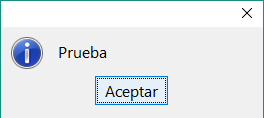
Inputbox:
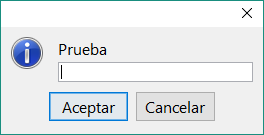
Confirm Dialog:
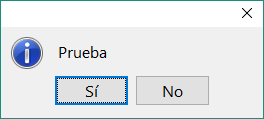
File chooser:
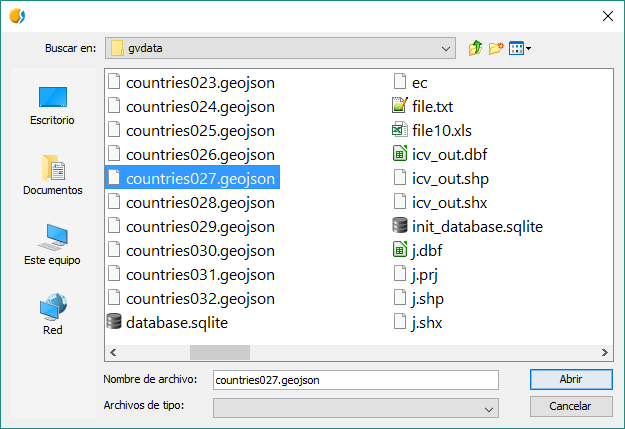
File chooser with multiselection:
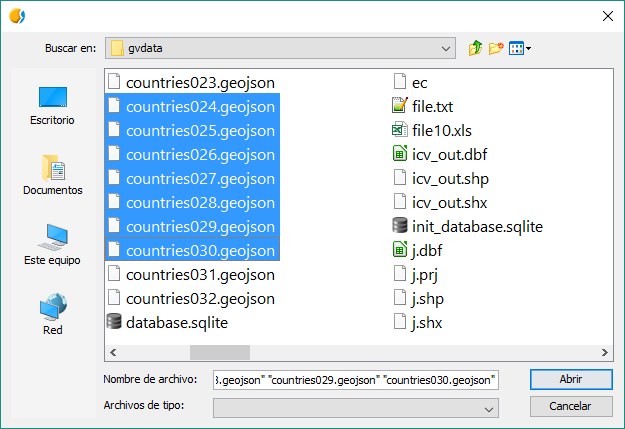
Open file dialog:
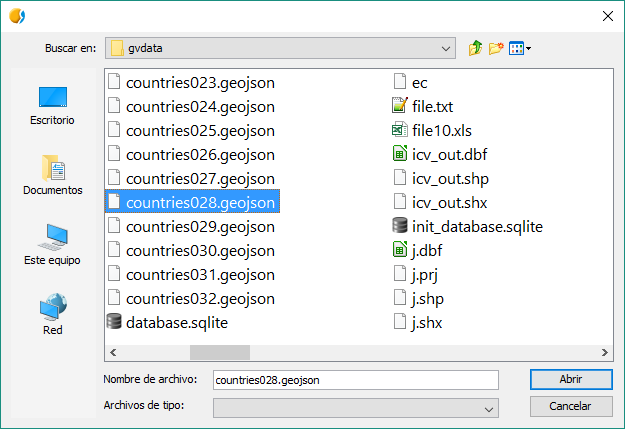
Open folder dialog:
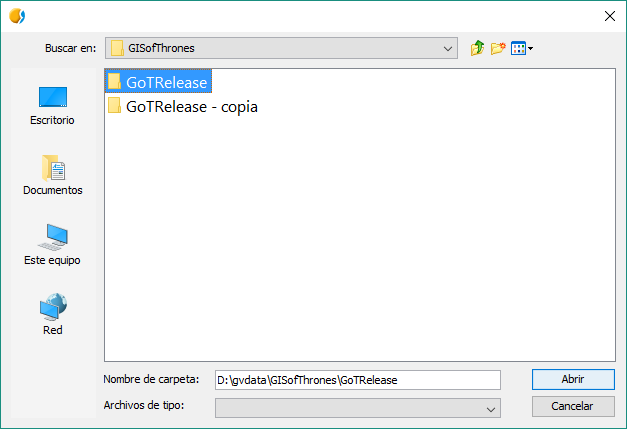
Save file dialog:
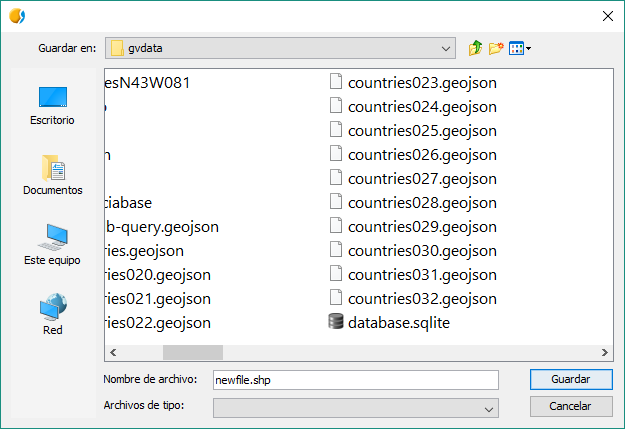
Console output:
msgbox: None
inputbox:
confirmDialog: 0
filechooser: D:\gvdata\countries027.geojson
filechooser: [u'D:\\gvdata\\countries024.geojson', u'D:\\gvdata\\countries025.geojson', u'D:\\gvdata\\countries026.geojson',
u'D:\\gvdata\\countries027.geojson', u'D:\\gvdata\\countries028.geojson', u'D:\\gvdata\\countries029.geojson',
u'D:\\gvdata\\countries030.geojson']
openFileDialog: [u'D:\\gvdata\\countries028.geojson']
openFolderDialog: [u'D:\\gvdata\\GISofThrones\\GoTRelease']
saveFileDialog: [u'D:\\gvdata\\newfile.shp']